Mastering the Art of Writing Clean and Maintainable JavaScript Code
Elevate Your JavaScript Skills by Embracing Clean Code Practices
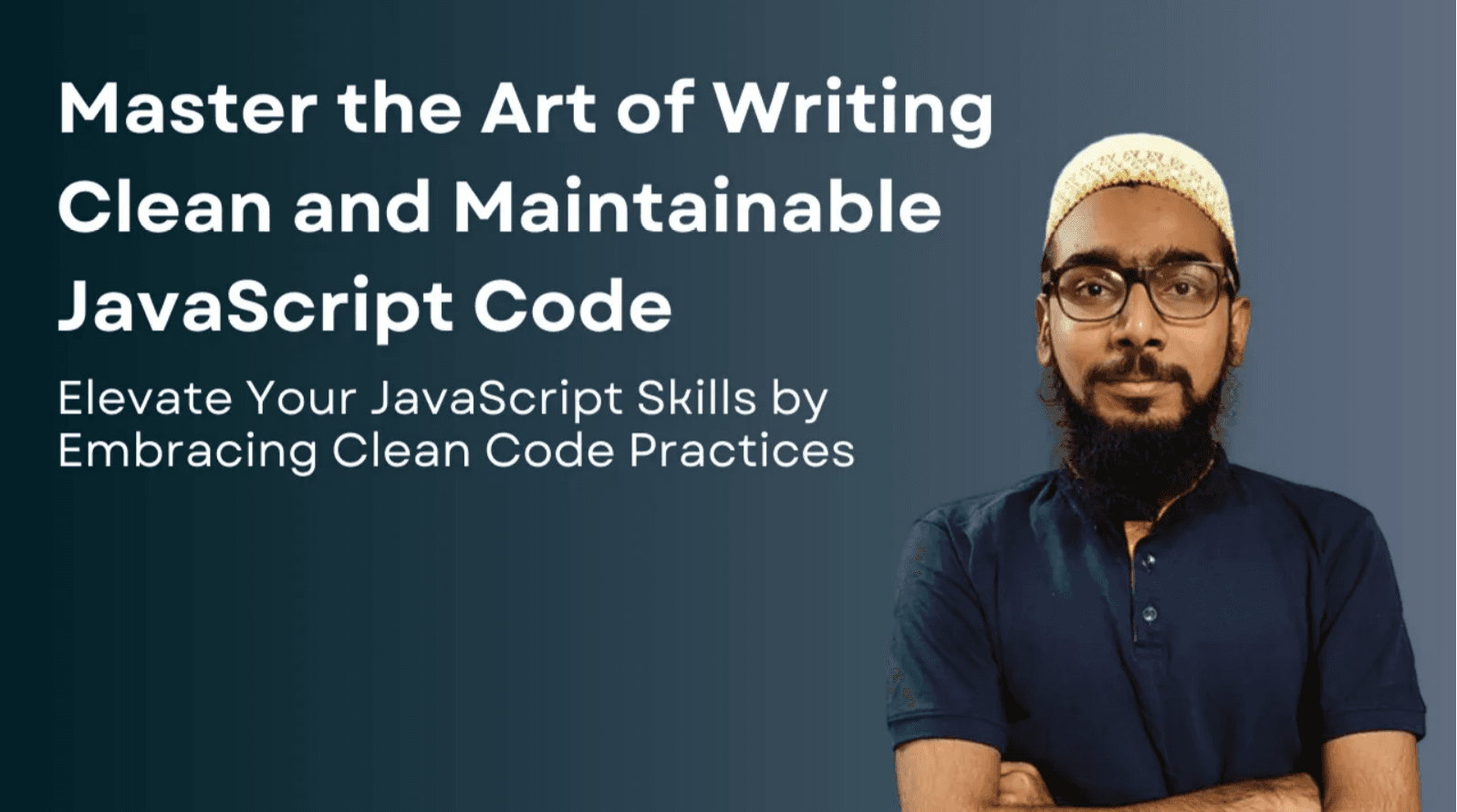
In today’s fast-paced development industry, creating clean and maintainable JavaScript is not a luxury, but a requirement. Whether you are a single developer or part of a large team, maintainable code facilitates communication, lowers defects, and ensures scalability. Mastering the skill of producing clean code is essential for true software development success.
Why Clean Code Matters
Clean code is more than simply aesthetically pleasing; it has a direct impact on the success and longevity of your project. Clean and maintainable JavaScript ensures the following:
Ease of collaboration: Collaboration is made easier when the code is well-structured. Lower bug counts: Readable code makes it easier to find and fix bugs. Scalability: Modular and well-organized code may be quickly expanded as your project grows. Improved performance: Clean code frequently leads to greater optimization, which means faster execution.
Let’s look at some essential ideas for building clean, maintainable JavaScript code.
- Use consistent naming conventions.
Choosing sensible names for variables, functions, and classes is the cornerstone of clean code. Follow conventions like camelCase for variables and functions, and PascalCase for class names.
Bad example:
const dt = new Date();
const x = calculateTotal(a, b);
Good example:
const currentDate = new Date();
const totalAmount = calculateTotal(price, quantity);
- Write small and focused functions.
Functions should ideally accomplish a single purpose. If your function performs many tasks, it becomes more difficult to test and maintain. Small, modular functions encourage code reuse and facilitate debugging.
Bad example:
function processOrder(order) {
validateOrder(order);
saveOrderToDatabase(order);
sendEmailConfirmation(order);
}
Good example:
function processOrder(order) {
validateOrder(order);
saveOrder(order);
sendConfirmationEmail(order);
}
function validateOrder(order) { /* ... */ }
function saveOrder(order) { /* ... */ }
function sendConfirmationEmail(order) { /* ... */ }
- Avoid deep nesting. Deeply nested code can be difficult to follow and maintain. Break down complex logic into smaller functions to avoid excessive nesting.
Bad example:
if (user) {
if (user.isAdmin) {
if (hasPermission(user)) {
// do something
}
}
}
Good example:
if (isAdminUser(user) && hasPermission(user)) {
// do something
}
function isAdminUser(user) {
return user && user.isAdmin;
}
- Use ES6+ features. Wisely JavaScript has developed over time, and new features might help you write cleaner, more concise code. Use arrow functions, destructuring, and template literals to their full potential.
Bad example:
function greet(name) {
return 'Hello, ' + name + '!';
}
Good example:
const greet = name => `Hello, ${name}!`;
- Embrace DRY (Do Not Repeat Yourself). Duplication of code indicates that your code may be more difficult to maintain. Always try to restructure repetitive functionality into reusable functions or components.
Bad example:
const userGreeting = `Hello, ${user.name}!`;
const adminGreeting = `Hello, ${admin.name}!`;
Good example:
const greetUser = (role, name) => `Hello, ${role.name}!`;
- Comment wisely. Comments should not describe what the code does; clean code should be self-explanatory. Instead, use comments to clarify why specific decisions were made, especially if they are not evident.
Bad example:
// increment x
x++;
Good example:
// Using a global counter to track user sessions.
sessionCounter.increment();
-
Create meaningful tests. Unit tests serve an important part in guaranteeing code maintainability. They help you ensure that your code works as expected and make reworking safer. Jest and Mocha are great for testing JavaScript code.
-
Use Linting Tools. JavaScript linting tools, such as ESLint, help maintain a uniform style throughout your codebase and identify possible flaws before they become problems. It’s a good idea to configure ESLint with rigorous rule sets for each project.
-
Break down large code bases. In huge projects, it is critical to divide your code into smaller modules. JavaScript modules (ES6 imports and exports) can be used to divide concerns while keeping the codebase reasonable.
-
Refactor often. Refactoring is the process of rearranging old code without modifying its functionality. Regular refactoring helps maintain your code clean and efficient. As you develop new features, don’t be afraid to revisit and improve existing code.
Conclusion
Writing clean and maintainable JavaScript code is a continuous process, not a one-time effort. It demands discipline and attention to detail, but the benefits are enormous: a more efficient, scalable, and entertaining codebase. By adhering to these principles, you will not only write better JavaScript, but also create a healthier development environment for yourself and your team.