TypeScript: The Essential Tool for Modern JavaScript Development
Unlocking the Power of TypeScript for Cleaner, More Reliable Code
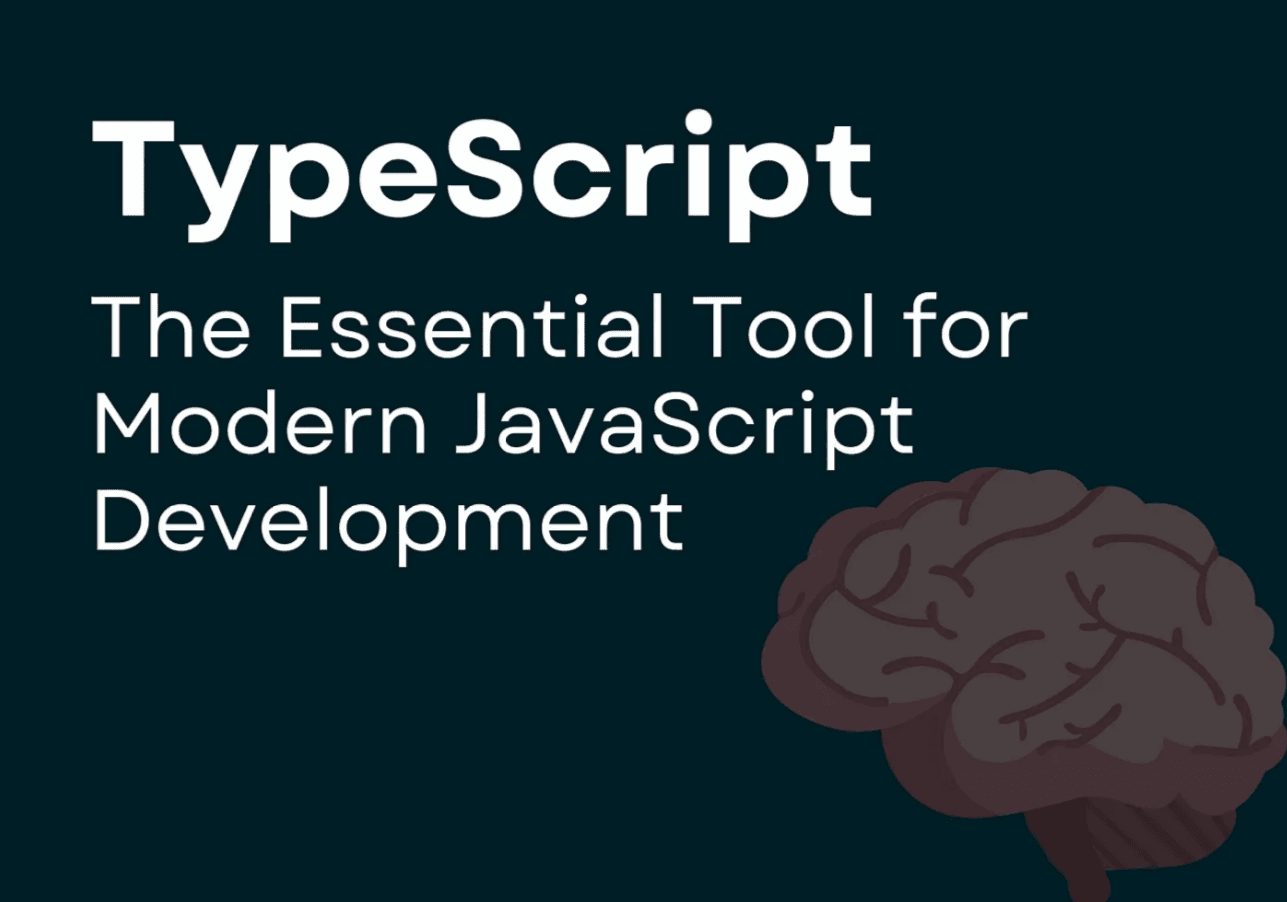
Efficiency and dependability are crucial in the quick-paced field of web development. As developers, we’re always looking for tools that will improve the robustness of our code while still making it elegant and simple to maintain. A clever and elegant answer is provided by TypeScript, a language that combines the rigor of static typing with the flexibility of JavaScript.
TypeScript: What Is It?
Microsoft developed TypeScript, which is a superset of JavaScript that includes static types. This makes your code more predictable and debug-friendly by allowing you to define the types of variables, functions, and objects. Variables in JavaScript are dynamically typed, meaning they can change types; in contrast, TypeScript enforces types, allowing problems to be discovered early in the development process.
The Reasons TypeScript Is Not Just a Superset
Although TypeScript appears to be merely another layer of complexity at first, it actually has a number of advantages that speed up development, that include:
Improved Code Quality: TypeScript’s static typing allows programmers to identify possible mistakes early in the development process, as opposed to at runtime. A more seamless development process and fewer bugs result from this.
Better Developer Experience: TypeScript’s strong type inference makes it possible for the language to comprehend types even in cases when they aren’t defined explicitly, which facilitates reading and writing code.
Improved Cooperation: TypeScript functions as a self-documenting code format in larger organizations. Without having to sift through documentation or rely only on intuition, developers may determine what a function or variable is intended to do.
Scalability: Keeping up with the codebase gets harder as projects get bigger. Because of TypeScript’s disciplined methodology, scalable and maintainable code is guaranteed to last as the project grows.
Smooth Integration with JavaScript: TypeScript code can be used in any environment that supports JavaScript because it compiles to ordinary JavaScript. One by one, you can convert files in your current JavaScript projects to incorporate TypeScript progressively.
TypeScript in Action: Real-World Examples
Asana, Slack, Airbnb, and many more top internet businesses have all embraced TypeScript. These businesses use TypeScript to decrease defects, expedite development, and increase the dependability of their codebase.
For instance, TypeScript is the primary language of the well-known front-end framework Angular, demonstrating how TypeScript can be used to create intricate, scalable applications.
How to Get Started with TypeScript
Getting started with TypeScript is simple. Here’s a basic example:
function add(a: number, b: number): number {
return a + b;
}
let sum = add(5, 10);
console.log(sum); // Outputs 15
In this example, the function add takes two numbers as arguments and returns a number. TypeScript checks that only numbers are passed to the function, ensuring that the function behaves as expected.
To start using TypeScript in your project:
- Install TypeScript using npm:
npm install -g typescript
- Compile a TypeScript file to JavaScript:
tsc filename.ts
- Integrate TypeScript with modern frameworks like Angular, React, or Node.js to leverage its full potential.
To sum up
TypeScript is more than just a JavaScript type addition tool. It’s an architectural change that gives your code scalability, safety, and structure. Developers may write cleaner, more dependable code, which reduces defects and speeds up development cycles, by embracing TypeScript. To put it briefly, TypeScript is a crucial component of any current developer’s toolset since it is the ideal fusion of intelligence and style.
“TypeScript is the bridge that connects JavaScript’s dynamic nature with the stability of static typing — empowering developers to write code that’s as elegant as it is efficient.” — Burhanuddin Mulla Hamzabhai