Mastering Async/Await in JavaScript: Simplifying Asynchronous Code
Learn how to streamline your asynchronous JavaScript code with Async/Await, making it more readable, maintainable, and efficient.
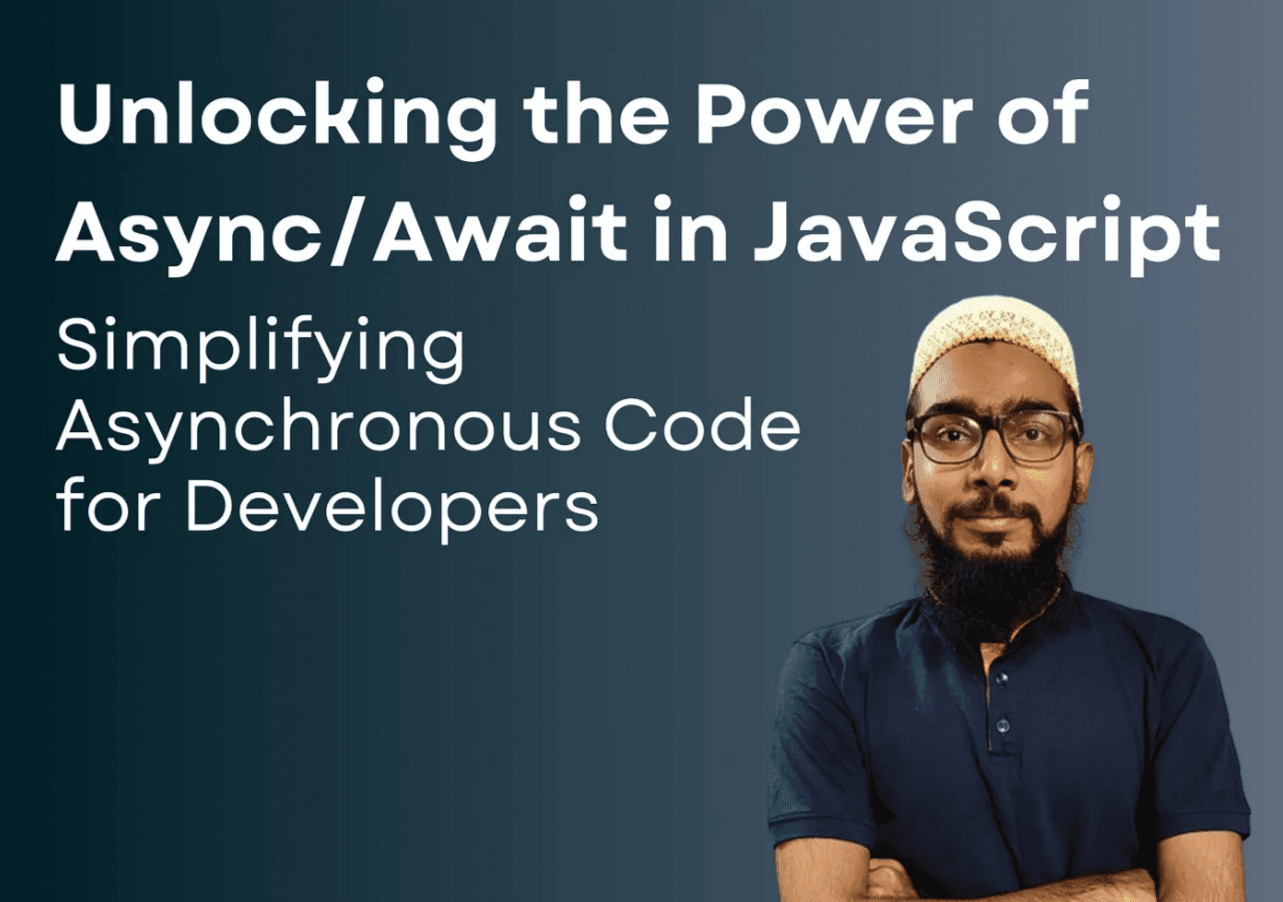
Handling asynchronous activities in JavaScript can quickly become complex and difficult to manage. Traditionally, callbacks and promises were the go-to solutions, but they came with their own set of issues, such as callback hell and promise chaining. Async/Await is a current way for simplifying asynchronous code, making it more legible and maintainable.
The Need for Async/Await.
Before delving into the complex workings of Async/Await, it’s important to understand what problem it solves. Asynchronous code is critical in JavaScript, particularly when dealing with tasks like as API calls, file I/O, and timers. Promises enhanced the way we handled these processes by providing a more controllable structure. However, promise chains may still grow complicated.
To solve these complications, ES2017 (ECMAScript 8) includes async/await, which allows developers to write asynchronous code that appears and performs like synchronous code.
Understanding Async/Await.
Async/Await is fundamentally based on promises. The async keyword defines an asynchronous function, and the await keyword can be used within this function to suspend execution until a promise is resolved or refused.
Here’s a simple example to illustrate:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
In this example, the fetchData function is declared async, indicating that it is asynchronous. Inside the function, the await keyword is used to wait for the fetch promise to resolve before proceeding to the next line of code. If the promise is refused, the catch block addresses the error, which prevents further promise rejections.
Advantages of Async/Await
Improved Readability: Improved code readability is the primary benefit. It removes the requirement for chaining.then() calls make the code appear cleaner and easier to grasp.
Error Handling: Async/Await simplifies error handling, making it equivalent to synchronous programming. You can handle errors using try/catch blocks, which is more straightforward than dealing with errors directly.Catch() in promise chains.
Synchronous-Like Flow: Despite being asynchronous, the flow of code written with Async/Await appears synchronous, making it easier to reason about, particularly for those unfamiliar with asynchronous programming.
Easier Debugging: Debugging Async/Await code is often easier than dealing with promises. Stepping through the code with a debugger is easier because it appears to be synchronous.
Real-World Example: Sequential vs. Concurrent Execution
Let’s take a practical example of fetching data from multiple APIs sequentially and concurrently to see how Async/Await can be utilized effectively.
Sequential Execution:
async function fetchSequentially() {
const data1 = await fetch('https://api.example.com/data1');
const data2 = await fetch('https://api.example.com/data2');
return [await data1.json(), await data2.json()];
}
Concurrent Execution:
async function fetchConcurrently() {
const [data1, data2] = await Promise.all([
fetch('https://api.example.com/data1'),
fetch('https://api.example.com/data2')
]);
return [await data1.json(), await data2.json()];
}
In the sequential example, each request waits for the preceding one to finish. This can be slow if the operations are independent. In contrast, the concurrent example initiates both requests at the same time, considerably enhancing performance when the actions are independent of one another.
Common Pitfalls and Best Practices
While Async/Await makes asynchronous coding easier, there are certain frequent dangers to be mindful of:
Forgetting await: If you forget to use await before a promise, the function will return a promise rather than the resolved value, which may result in unexpected behavior.
Nested Asynchronous Calls: Avoid highly nested asynchronous functions, as they can still result in complex code. Refactor wherever possible to keep the structure flat.
Error Handling: Always use try/catch to avoid unhandled promise rejections, especially in production code.
Best practices:
- To execute independent asynchronous activities in parallel, use Promise.all().
- Keep your asynchronous code flat and avoid extensive nesting.
- Always use try/catch to manage errors in your asynchronous functions.
Conclusion
Async/Await is an extremely useful technique in the JavaScript developer’s toolkit. The ability to write asynchronous code that appears and feels like synchronous code enhances readability, maintainability, and overall developer experience. Mastering Async/Await allows you to write code that is clearer, more efficient, and easier to debug, resulting in more resilient JavaScript applications.
“Writing asynchronous code doesn’t have to be a struggle. With Async/Await, you can make your code as smooth as a well-composed melody.” — Burhanuddin Mulla Hamzabhai